Winxbet, Avrupa’nın en büyük ve en saygın lisanslı bahis platformlarından biri olarak Türkiye’deki kullanıcılarla 10 yılı aşkın deneyimini paylaşıyor. Spor bahisleri ve casino oyunları konusunda üst düzey hizmet sunan bu site, Türkiye’nin standartlarının ötesinde bir performans sergiliyor ve kesintisiz olarak uluslararası bir hizmet sunuyor. İngiltere merkezli olan Winxbet, Avrupa’nın altı farklı ülkesinde bahis faaliyetlerini sürdürerek kullanıcılara uluslararası standartlarda güvenilir bir bahis ortamı sunuyor.
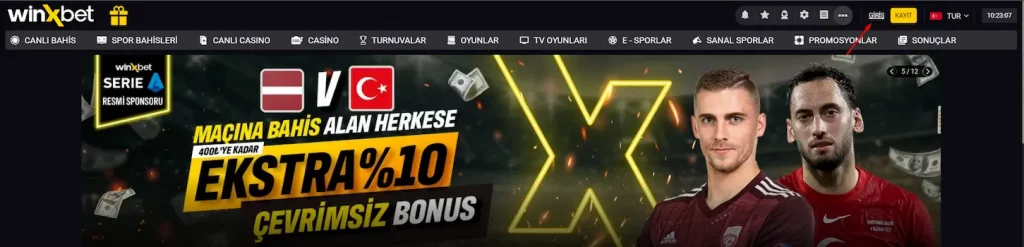
Winxbet Güncel Giriş
Ülkemizdeki online bahis sitelerinin erişimine getirilen kısıtlamalar, bahis severlerin ve oyuncuların deneyimlerini olumsuz etkileyebiliyor. Ancak, bu tür engellemelere çözüm sunan siteler, kullanıcıların sorunsuzca bahis yapmalarını ve oyunlarını oynamalarını sağlıyor. Winxbet de, kullanıcılarına kesintisiz bir bahis deneyimi sunabilmek adına sürekli güncellenen giriş adresleriyle öne çıkıyor.
%100 Spor/Casino Bonusu – 2000₺ Hoşgeldin Bonusu İçin Buraya Tıklayın!
Online bahis sitelerinin ülkemizdeki engellemelere maruz kalmasının sebepleri arasında birkaç faktör öne çıkıyor:
Lisans Konusu: Birçok online bahis sitesi, Türkiye’de geçerli olmayan yabancı lisanslara sahip olabilir. Bu durum, sitelerin kaçak olarak kabul edilmesine yol açabilir.
Yasal Düzenlemeler: Türkiye’de sadece devlete ait İddaa sistemi yasal olarak tanınırken, diğer online bahis siteleri yasal düzenlemelere uymadığı için kaçak olarak değerlendirilebilir.
Vergi Sorunu: Türkiye’de lisans alamayan online bahis şirketleri, vergilerini yurt dışında ödemek durumunda kalabilirler. Bu durum, Türkiye’deki gelirlerin denetlenmesini ve vergilendirilmesini zorlaştırabilir.
Casino Oyunları: Türkiye’de casino oyunları yasaklanmış olmasına rağmen, bazı online bahis siteleri bu oyunları sunabilir. Bu durum da sitelerin engellenmesinin bir sebebi olabilir.
Winxbet, bu tür engellemelere karşı çözümler üreten ve kullanıcılarının mağduriyet yaşamamasını sağlayan bir platform olarak öne çıkıyor. Sürekli güncellenen giriş adresleriyle kullanıcılarına sorunsuz bir deneyim sunmayı amaçlıyor. Güvenilir ve kullanıcı dostu bir ortam sağlama konusunda önemli bir yer edinen Winxbet, bahis ve casino tutkunları için güçlü bir alternatif sunuyor. Bu sayede, bahis tutkunları Winxbet üzerinden keyifli bir deneyim yaşayabiliyor.
WinXbet Yeni Giriş Adresi
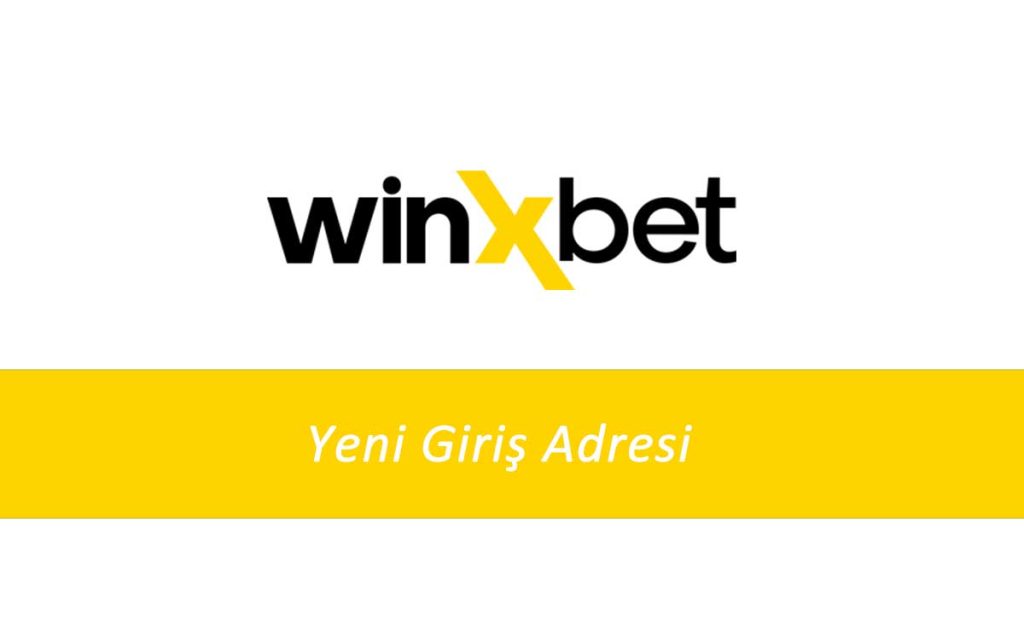
Son zamanlarda WinXbet’in yeni giriş adresi oldukça merak edilen bir konu haline gelmiştir. Bu yüzden, sadece erişim bilgilerine değil, aynı zamanda kullanıcı yorumlarına da önem vermek faydalı olabilir. Çünkü bu firmanın kullanıcıları tarafından yapılan yorumların neredeyse tamamı olumlu yöndedir. Bu durum, başarılı bir bahis firması arayanların genellikle bu siteye üye olmalarına sebep olmaktadır.
Winxbet Mobil Giriş Adresi
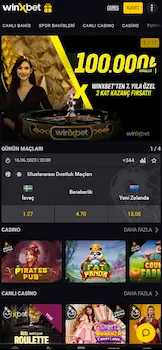
Winxbet’in mobil giriş adresi, bahsetmek istediğimiz konulardan biri. Bu adres sayesinde mobil cihazlarınızdan siteye erişebilir ve işlemlerinizi hızlıca gerçekleştirebilirsiniz. Çünkü her zaman evde olmayabilir veya bilgisayarınız yanınızda olmayabilir. Mobil giriş butonu, bu gibi durumlarda kullanıcıları siteye yönlendirerek pratik bir çözüm sunuyor.
Winxbet, mobil uyumlu web sitesi desteğiyle kullanıcılarına büyük bir kolaylık sağlıyor. Şimdi araştırmalarınızı yapabilir, isterseniz bahislerinizi alabilirsiniz. Winxbet gibi adresler her zaman önemli ve ihtiyaçlarımızı en iyi şekilde karşıladıklarını düşünüyoruz.
Winxbet mobil girişi, cep telefonlarınızı gerçek bir kumarhane gibi kullanmanızı sağlayacak. Firmanın sektörün ihtiyaçlarını belirleyen profesyonel kadrosuyla memnuniyetinizi sağlamayı hedefliyor. Diğer tüm detayları ise siteyi ziyaret ettiğinizde daha net bir şekilde görebilirsiniz.
Winxbet Giriş Nasıl Yapılır?
Elbette, Winxbet’e adım adım giriş yapmak için şu adımları takip edebilirsiniz:
- İnternet tarayıcınızı açın ve arama motoruna “Winxbet” terimini aratın.
- Çıkan sonuçlardan resmi Winxbet sitesini bulun ve tıklayın.
- Siteye girdiğinizde karşınıza genellikle ana sayfa çıkacaktır. Eğer daha önce üye olduysanız, sağ üst köşede “Giriş” veya “Üye Girişi” gibi bir seçenek olacaktır. Buraya tıklayın.
- Kullanıcı adı ve şifrenizi girerek giriş yapabilirsiniz. Eğer daha önce üye olmadıysanız, genellikle “Kayıt Ol” veya “Üye Ol” gibi bir buton bulunur. Bu seçeneği tıklayarak gerekli bilgileri doldurarak kayıt işlemini tamamlayabilirsiniz.
- Üye girişi yaptıktan sonra, hesabınıza erişim sağlayabilir ve Winxbet’in sunmuş olduğu hizmetleri kullanmaya başlayabilirsiniz.
Giriş işlemi için kullanıcı adı ve şifrenizin doğru ve güncel olması önemlidir. Ayrıca, resmi ve güvenilir olan Winxbet sitesine giriş yapmanız da önemlidir, dolayısıyla doğru adrese yönlendiğinizden emin olun.

Winxbet Bonusları
Winxbet’in bonusları gerçekten iddialı ve oyunculara çeşitli kazançlar sağlayan kampanyalar sunuyor. İşte Winxbet’in bonuslarına ilişkin övgü dolu bazı özellikler:
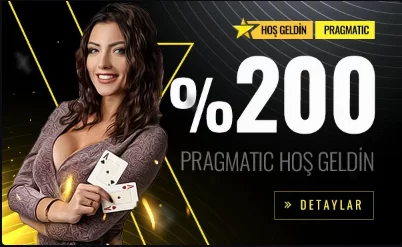
Hoş Geldin Bonusu: Yeni üyelere özel sunulan bu bonus, ilk bir veya üç yatırımlarını katlamalarına olanak tanır. Genellikle ilk yatırımın bir yüzdesi şeklinde verilir ve oyuncuların daha fazla bahis yapmalarını ve kazanç elde etmelerini sağlar.
Yatırım Bonusları: Winxbet, belirli yatırım yöntemlerini kullanan oyunculara özel bonuslar sunar. Örneğin, belirli bir e-cüzdan veya kripto para kullanımında ekstra bonuslar kazanılabilir. Bu, oyuncuların farklı ödeme seçeneklerini denemelerine teşvik eder.
Kayıp Bonusları: Winxbet, oyuncuların kayıplarını telafi etmelerine yardımcı olmak için kayıp bonusları sunar. Belirli bir süre içinde yaşanan kayıpların bir kısmını geri alma imkanı sunarak oyuncuların motivasyonunu artırır.
Winxbet’in bonusları, kullanıcıların oyun keyfini artırmak ve daha fazla kazanç elde etmelerini sağlamak adına çeşitli fırsatlar sunar. Bu bonuslar, kullanıcıların deneyimlerini daha eğlenceli hale getirir ve daha fazla kazanç sağlamalarına yardımcı olur.
Winxbet Güvenilir Mi?
Winxbet’in güvenilirliği konusunda bir araştırma yapalım. Site, güçlü altyapısıyla her kullanıcıya beklentilerine uygun hizmet sunuyor. 8048/JAZ lisansıyla faaliyet gösteren firma, Curacao Devleti tarafından yetkilendirilmiş durumda. WB Ventures N.V. anonim şirketi tarafından desteklenen sitenin altyapısı, özgün kalitesiyle kullanıcıların memnuniyetini sağlıyor. Bu, sitenin güvenilirliği açısından önemli bir gösterge. Ancak gerçek bir değerlendirme için kullanıcıların deneyimleri ve incelemeleri önemli. Soruya vereceğimiz cevap, şu an için olumlu yönlü oluyor.